Category Archives: Programming
Creating a Plasma effect for the Arduino and the Sparkfun LED tile
After having discovered the Arduino and before attacking the network extension I wanted to create something fun with the board and the 8 by 8 LED tile.
So I came with the idea of using one old school plasma effect of ArKaos VJ and porting it to the Arduino.
Here is the result:
Basically there are 2 textures pre-computed at startup. They are moved on a circular motion.
The 2 textures are moved and summed and you obtain an height value for each pixel. To get a nice effect you then go through a color table that is also pre-computed at startup.
To have a nice rotation effect you shift the color table at each generation of a new image.
At the end of the video you have another simple loop I did to test my setup.
Here is the plasma code for the Arduino:
//
// Plasma generation for the Arduino and a RGB Serial Backpack Matrix from SparkFun Electronics
// Marco Hinic, built on top of the code of Ryan Owens
//
//Define the SPI Pin Numbers
#define DATAOUT 11//MOSI
#define DATAIN 12//MISO
#define SPICLOCK 13//sck
#define SLAVESELECT 10//ss
void LED_Setup()
{
//SPI Bus setup
SPCR = (1<
// 16 by 16 -> 256 bytes
// color table 256 bytes
//
#define PLASMA_W 8
#define PLASMA_H 8
#define TABLE_W 16
#define TABLE_H 16
unsigned char gPlasma[PLASMA_W*PLASMA_H];
unsigned char gTable1[TABLE_W*TABLE_H];
unsigned char gTable2[TABLE_W*TABLE_H];
unsigned char gColorTable[256];
float gCircle1, gCircle2, gCircle3, gCircle4, gCircle5, gCircle6, gCircle7, gCircle8;
int gRoll;
void Plasma_CalcTable1 ()
{
for (int i=0; i< TABLE_H; i++)
{
for (int j=0; j< TABLE_W; j++)
{
int index = (i*TABLE_W)+j;
gTable1[index] = (unsigned char) ((sqrt(16.0+(PLASMA_H-i)*(PLASMA_H-i)+(PLASMA_W-j)*(PLASMA_W-j))-4) *5 );
}
}
}
void Plasma_CalcTable2 ()
{
for (int i=0; i< TABLE_H; i++)
{
for (int j=0; j< TABLE_W; j++)
{
int index = (i*TABLE_W)+j;
float temp = sqrt(16.0+(PLASMA_H-i)*(PLASMA_H-i)+(PLASMA_W-j)*(PLASMA_W-j))-4;
gTable2[index] = (sin(temp/9.5)+1)*90;
}
}
}
void Plasma_SetColor (int index, unsigned char red, unsigned char green, unsigned char blue)
{
unsigned char new_color = (red & 0xE0) + ((green & 0xE0) >> 3) + ((blue & 0xC0) >> 6);
gColorTable [index] = new_color;
}
double gRed, gGreen, gBlue;
#define color(u,a) (cos((u)+(a))+1)*127
void BuildColorTable()
{
double u;
int i;
for (i=0; i<256; i++)
{
u=2*PI/256*i;
Plasma_SetColor(i,color(u,gRed),color(u,gGreen),color(u,gBlue));
}
gRed+=0.05;
gGreen-=0.05;
gBlue+=0.1;
}
void Plasma_Setup ()
{
gCircle1 = 0;
gCircle2 = 0;
gCircle3 = 0;
gCircle4 = 0;
gCircle5 = 0;
gCircle6 = 0;
gCircle7 = 0;
gCircle8 = 0;
gRoll = 0;
for (int i=0; i
First contact with the Arduino
I bought a small Arduino board that has a small CPU to prototype some ideas about driving LEDs.
Here is the first test, it’s was quick to have something blinking!
I want to use that as a prototyping platform to develop a protocol to drive LEDs in a simpler way.
Today you drive LED or with DMX or with video converters. DMX is cool and inexpensive but difficult to setup and video converters are very expansive.
There should be a way to connect LEDs to a computer and having them recognized automatically by a VJ software…
If you are curious to see on how simple the code is, here it is:
// Writing to the RGB Serial Backpack Matrix from SparkFun Electronics
// Marco Hinic, built on top of the code of Ryan Owens
//
//Define the SPI Pin Numbers
#define DATAOUT 11//MOSI
#define DATAIN 12//MISO
#define SPICLOCK 13//sck
#define SLAVESELECT 10//ss
//Define the variables we’ll need later in the program
char color_buffer [64];
void setup()
{
//SPI Bus setup
SPCR = (1<
An application to create time lapse: Lapseler
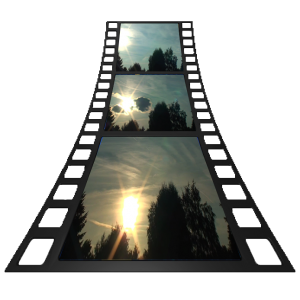
Lapseler
After a few months of tweaking the code I released today Lapseler.
Lapselser is an application that can take pictures from any QuickTime supported devices and compile them in a movie.
Because the compression library is from FFmpeg it can generate QuickTime files but also flash videos.
The motivation to work on this application is both that I am interested into time lapse since I saw Koyaanisqatsi many years ago and I am learning several interfaces of Mac OS X / cocoa right now.
Lapseler is available on my web site:
fraktus.com/lapseler/
Leave me a note if you make some use of it.
The app is completely free and has no limitation, so you can use it to make cool videos for whatever purpose.
Easily converting an image into C++ / Obj C code..
If you need a way to convert simply a small image into C++ / Obj C code that can be compiled by GCC or any other modern compiler there are a few utilities out there. In my case I was not happy with the results, so I wrote a few lines of code to do this.
What I needed is a simple code that fill an array of char that can then be used by OpenGL or custom pixel processing code. It’s so easy to load an image into an NSBitmapImageRep. Once you have this there are a few accessor like pixelsWide, bitmapData … that lets you play with the pixels and it’s then very easy to generate some text that will be C++ / Obj C compliant.
Here is a small 16 by 16 image converted into code with my utility:
int gImageWidth = 16;
int gImageHeight = 16;
int gImageBits = 24;
unsigned char gImagePixels[] = {
// line 0
0xf2,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,0xf1,
0xf1,0xf1,0xf1,0xf0,0xf0,0xf0,0xf0,0xf0,0xf0,0xf0,0xf0,0xf0,
0xf0,0xf0,0xf0,0xf0,0xf0,0xf0,0xef,0xf0,0xf0,0xef,0xef,0xef,
0xef,0xef,0xef,0xef,0xef,0xef,0xef,0xef,0xef,0xee,0xee,0xee,
// line 1
0xe5,0xe5,0xe5,0xe5,0xe5,0xe5,0xe4,0xe4,0xe4,0xe4,0xe4,0xe4,
0xe4,0xe4,0xe4,0xe4,0xe4,0xe4,0xe4,0xe3,0xe4,0xe3,0xe3,0xe3,
0xe3,0xe3,0xe3,0xe3,0xe3,0xe3,0xe3,0xe3,0xe3,0xe2,0xe2,0xe2,
0xe2,0xe2,0xe2,0xe2,0xe2,0xe2,0xe2,0xe2,0xe2,0xe1,0xe1,0xe1,
// line 2
0xd7,0xd7,0xd7,0xd7,0xd7,0xd7,0xd7,0xd7,0xd7,0xd6,0xd6,0xd6,
0xd6,0xd6,0xd6,0xd6,0xd6,0xd6,0xd6,0xd6,0xd6,0xd5,0xd5,0xd5,
0xd5,0xd5,0xd5,0xd5,0xd5,0xd5,0xd4,0xd5,0xd5,0xd4,0xd4,0xd4,
0xd4,0xd4,0xd4,0xd4,0xd4,0xd4,0xd3,0xd3,0xd4,0xd3,0xd3,0xd3,
…
And here is the code of the small command line utility:
#import Cocoa/Cocoa.h
int main (int argc, const char * argv[])
{
if (argc >=3)
{
NSAutoreleasePool *pool = [[NSAutoreleasePool alloc] init];
NSString * src_file = [[NSString alloc] initWithFormat: @”%s”, argv[1]];
NSData * raw = [NSData dataWithContentsOfFile: src_file];
if (raw)
{
NSBitmapImageRep *rep = [NSBitmapImageRep imageRepWithData: raw];
if (rep)
{
FILE * out = fopen (argv[2], “w”);
if (out)
{
int width = [rep pixelsWide];
int height = [rep pixelsHigh];
int bits = [rep bitsPerPixel];
int rowbytes = [rep bytesPerRow];
unsigned char * src = [rep bitmapData];
fprintf (out, “int gImageWidth = %ld;\n”, width);
fprintf (out, “int gImageHeight = %ld;\n”, height);
fprintf (out, “int gImageBits = %ld;\n”, bits);
fprintf (out, “unsigned char gImagePixels[] = {\n”);
for (int v=0; v